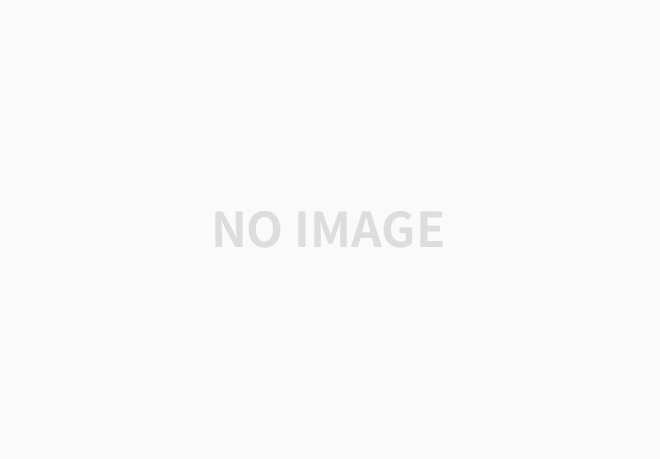
create view는 form은 있지만 object가 없고
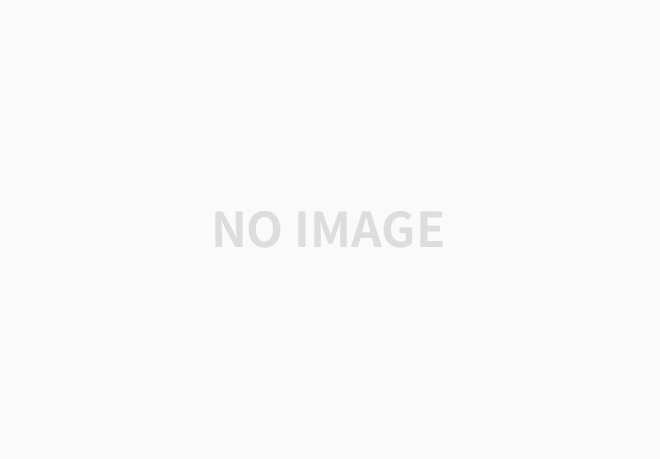
Detail view는 object가 있지만 form이 없다.
Detail view를 form과 같이 사용하고 싶다면? Mixin
detail view 안에 있었는데 comment form 밑에 달수 있게 됐다.
commentapp 구현사항
1. Create / Delete View
2. Success_url to related article
3. Model (article / writer / content / created_at)
1
python manage.py startapp commentapp
2
settings.py에 추가
3
platypus/urls.py 패스추가
from django.conf import settings
from django.conf.urls.static import static
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('accounts/', include('accountapp.urls')),
path('profiles/', include('profileapp.urls')),
path('articles/', include('articleapp.urls')),
path('comments/', include('commentapp.urls')),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
4
commentapp/urls.py 생성
from django.urls import path
from commentapp.views import CommentCreateView
app_name = 'commentapp'
urlpatterns = [
path('create/', CommentCreateView.as_view(), name='create'),
]
5
models.py
from django.contrib.auth.models import User
from django.db import models
# Create your models here.
from articleapp.models import Article
class Comment(models.Model):
article = models.ForeignKey(Article, on_delete=models.SET_NULL, null=True, related_name='comment')
writer = models.ForeignKey(User, on_delete=models.SET_NULL, null=True, related_name='comment')
comment = models.TextField(null=False)
create_at = models.DateTimeField(auto_now=True)
6
commentapp/forms.py
from django.forms import ModelForm
from commentapp.models import Comment
class CommentCreationForm(ModelForm):
class Meta:
model = Comment
fields = ['content']
7
python manage.py makemigrations
python manage.py migrate
8
views.py
from django.shortcuts import render
# Create your views here.
from django.urls import reverse
from django.views.generic import CreateView
from commentapp.forms import CommentCreationForm
from commentapp.models import Comment
class CommentCreateView(CreateView):
model = Comment
form_class = CommentCreationForm
template_name = 'commentapp/create.html'
def get_success_url(self):
return reverse('articleapp:detail',kwargs={'pk': self.object.article.pk})
9
commentapp/templates/commentapp/create.html
{% extends 'base.html'%}
{% load bootstrap4 %}
{% block content %}
<div style="text-align: center; max-width: 500px; margin: 4rem auto">
<div class="mb-4">
<h4>Comment Create</h4>
</div>
<form action="{% url 'commentapp:create' %}" method="post">
{% csrf_token %}
{% bootstrap_form form %}
<input type="submit" class="btn btn-dark rounded-pill col-6 mt-3">
<input type="hidden" name="article_pk" value="">
</form>
</div>
{% endblock %}
10
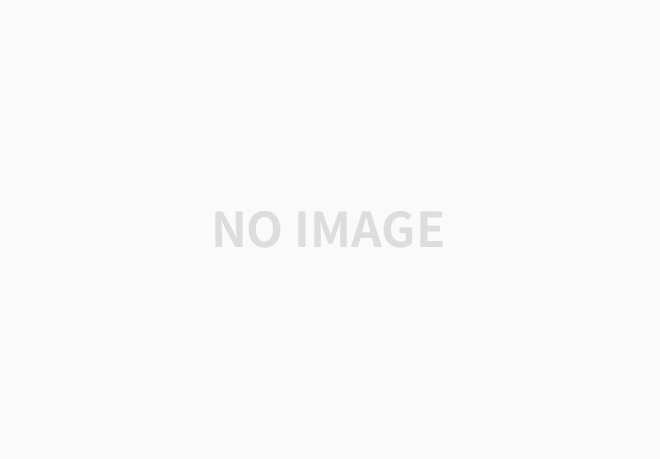
잘 나온다. 하지만 이렇게 코맨트를 따로 만들수 있는 페이지를 들어가는게 아니라 게시글 밑에 달기를 원한다. 이 레이아웃을 게시글 아래에 넣자. include 구문으로 가져오면 될것.
11
articleapp/detail.html
{% extends 'base.html' %}
{% block content %}
<div>
<div style="text-align: center; max-width: 700px; margin: 4rem auto;">
<h1>
{{ target_article.title }}
</h1>
<h5>
{{ target_article.writer.profile.nickname}}
</h5>
<hr>
<img style="width: 100%; border-radius: 1rem; margin: 2rem 0"
src="{{ target_article.image.url }}" alt="">
<p>
{{ target_article.content }}
</p>
{% if target_article.writer == user %}
<a href="{% url 'articleapp:update' pk=target_article.pk %}"
class="btn btn-primary rounded-pill col-3">
Update
</a>
<a href="{% url 'articleapp:delete' pk=target_article.pk %}"
class="btn btn-danger rounded-pill col-3">
Delete
</a>
{% endif %}
<hr>
{% include 'commentapp/create.html' with article=target_article %}
</div>
</div>
{% endblock %}
commentapp/create.html
{% load bootstrap4 %}
{% block content %}
<div style="text-align: center; max-width: 500px; margin: 4rem auto">
<div class="mb-4">
<h4>Comment Create</h4>
</div>
<form action="{% url 'commentapp:create' %}" method="post">
{% csrf_token %}
{% bootstrap_form form %}
<input type="submit" class="btn btn-dark rounded-pill col-6 mt-3">
<input type="hidden" name="article_pk" value="{{ article.pk }}">
</form>
</div>
{% endblock %}
12
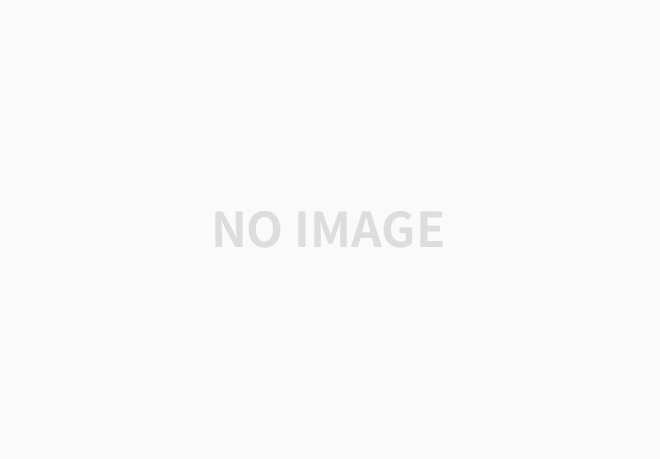
articleapp/views.py를 보면
class ArticleDetailView(DetailView):
model = Article
context_object_name = 'target_article'
template_name = 'articleapp/detail.html'
form mixin을 사용해야하는데 그 form이 아직 없다
class ArticleDetailView(DetailView, FormMixin):
model = Article
form_class = CommentCreationForm
context_object_name = 'target_article'
template_name = 'articleapp/detail.html'
13
commentapp/views.py
from django.shortcuts import render
# Create your views here.
from django.urls import reverse
from django.views.generic import CreateView
from articleapp.models import Article
from commentapp.forms import CommentCreationForm
from commentapp.models import Comment
class CommentCreateView(CreateView):
model = Comment
form_class = CommentCreationForm
template_name = 'commentapp/create.html'
def form_valid(self, form):
temp_comment = form.save(commit=False)
temp_comment.article = Article.objects.get(pk=self.request.POST['article_pk'])
temp_comment.writer = self.request.user
temp_comment.save()
return super().form_valid(form)
def get_success_url(self):
return reverse('articleapp:detail', kwargs={'pk': self.object.article.pk})
'웹 프로그래밍' 카테고리의 다른 글
[pinterest clone (32)] Projectapp 구현 (0) | 2021.05.02 |
---|---|
[pinterest clone (31)] 모바일 디버깅, 반응형 레이아웃 (0) | 2021.05.02 |
[pinterest clone (29)] ListView, Pagination 적용 (0) | 2021.05.01 |
[pinterest clone (28)] 문제사항 수정 (0) | 2021.05.01 |
[pinterest clone (27)] articleapp 구현 (0) | 2021.05.01 |